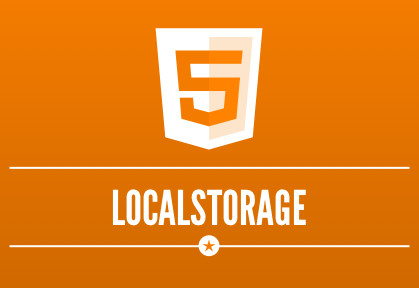
Pre fill form inputs with JavaScript from LocalStorage
- 4 minsOne of my long-term side projects is a community event website for my hometown of Penzance, called West Cornwall Events. At the time of writing it’s been online for almost six years, and over that time has had many regular event submissions from people in the local community. The latest developments on the site have been to the back-end, in an effort to automate as much of the day-to-day administration as possible. This required a ground up redevelopment of the event submission flow.
In recent months I’ve read allot about the usability and effectiveness of forms. One of the recurring themes is that the user should only have to fill in what is necessary and, if possible, fields should be prefilled. For this site I started by adding a drop down for existing venues, date and time pickers and visual prompts via placeholders and field highlighting upon error. After these early optimizations, and a look through stats related to past submissions, I found the following two things to be true.
- 70% of users made 2 or more submissions
- 37% of submissions came from just 5 people
I realised that I could quite easily speed up event submissions for these key people by pre-populating the users details fields. Whilst the application does track who submits which event, there are no user accounts as I didn’t want to put people off from submitting by increasing the time they needed to invest.
The Solution – LocalStorage & JavaScript
I decided on a simple solution using JavaScript and the LocalStorage API for storing the relevant information within the end users browser. The app I added this too uses jQuery, as does this tutorial.
Before we use the LocalStorage API we first need to test that it’s available. Following the principles of progressive enhancements, this form should function without the need for JavaScript or modern API’s. After getting inspiration (ahem) from the feature detection in Modenizr I used this function, which will return a boolean for use in an if statement later on.
Open up your JavaScript file and add the following function.
function lsTest(){
var test = 'test';
try {
localStorage.setItem(test, test);
localStorage.removeItem(test);
return true;
} catch(e) {
return false;
}
}
Here we’re trying to write and remove an item from LocalStorage. The function will return true if the catch block is not entered. Next we need to write a function to save the user’s details into LocalStorage. Add the following function to your js file.
function saveUserDetails() {
var user = {
'first': $('#user_event_first_name').val(),
'last': $('#user_event_last_name').val(),
'email': $('#user_event_user_email').val()
};
localStorage.setItem('wceUser', JSON.stringify(user));
}
The first few lines creates an object of the values the user entered into the appropriate inputs before saving the object to LocalStorage. As the object is a fairly simple one, I’ve used JSON.stringify
to convert the details to a string to allow easy conversion when retrieving back from storage.
Speaking of which we need another function to retrieve the users details and placing them back into the form.
function fetchUserDetails() {
var lsUser = localStorage.getItem('wceUser');
var user = JSON.parse(lsUser);
if (isRealValue(user)){
$('#user_event_first_name').val(user.first);
$('#user_event_last_name').val(user.last);
$('#user_event_user_email').val(user.email);
}
}
Here we fetch the item from storage, using the same key, and parse it back into a user object. The if statement checks that the stored values have successfully been converted back into an object (we’ll get to that). If it has we then insert the retrieved values back into the form. The following function is how we check that an object is actually an object, not undefined, by returning true or false.
function isRealValue(obj){
return obj && obj !== "null" && obj!== "undefined";
}
Simples. Now it’s time to put it all together. Within your document ready function add the following.
if(lsTest() === true){
if (localStorage.getItem("wceUser") !== null){
fetchUserDetails();
}
$("#submission-btn").hover(saveUserDetails);
}
We first wrap everything in our test of LocalStorage availability. If we have the power, the code will check that the appropriate key is stored locally, calling fetchUserDetails()
if it is. We then set up a listener on the submit button for the hover action. Whilst this does not give much time to perform the actual saving of user details (300ms ish on most devices), it should be enough. It’s also worth noting that there is no hover action on touch devices, although some will trigger the action before the click event. As this is an enhancement to the form, in this situation these two drawbacks do not present an issue.
Summary
That’s it. We’ve just saved form data to LocalStorage with appropriate checking of availability and parse success. Simple enhancements like this can greatly reduce drop off experienced during the completion of forms as part of your application.
If you liked this tutorial, can suggest improvements or just want to say thanks, get in touch on twitter at tonyedwardspz.